React
Building Scalable React Applications with Redux Toolkit
Learn how to manage complex application state effectively using Redux Toolkit in React applications.
Obinna Aguwa •
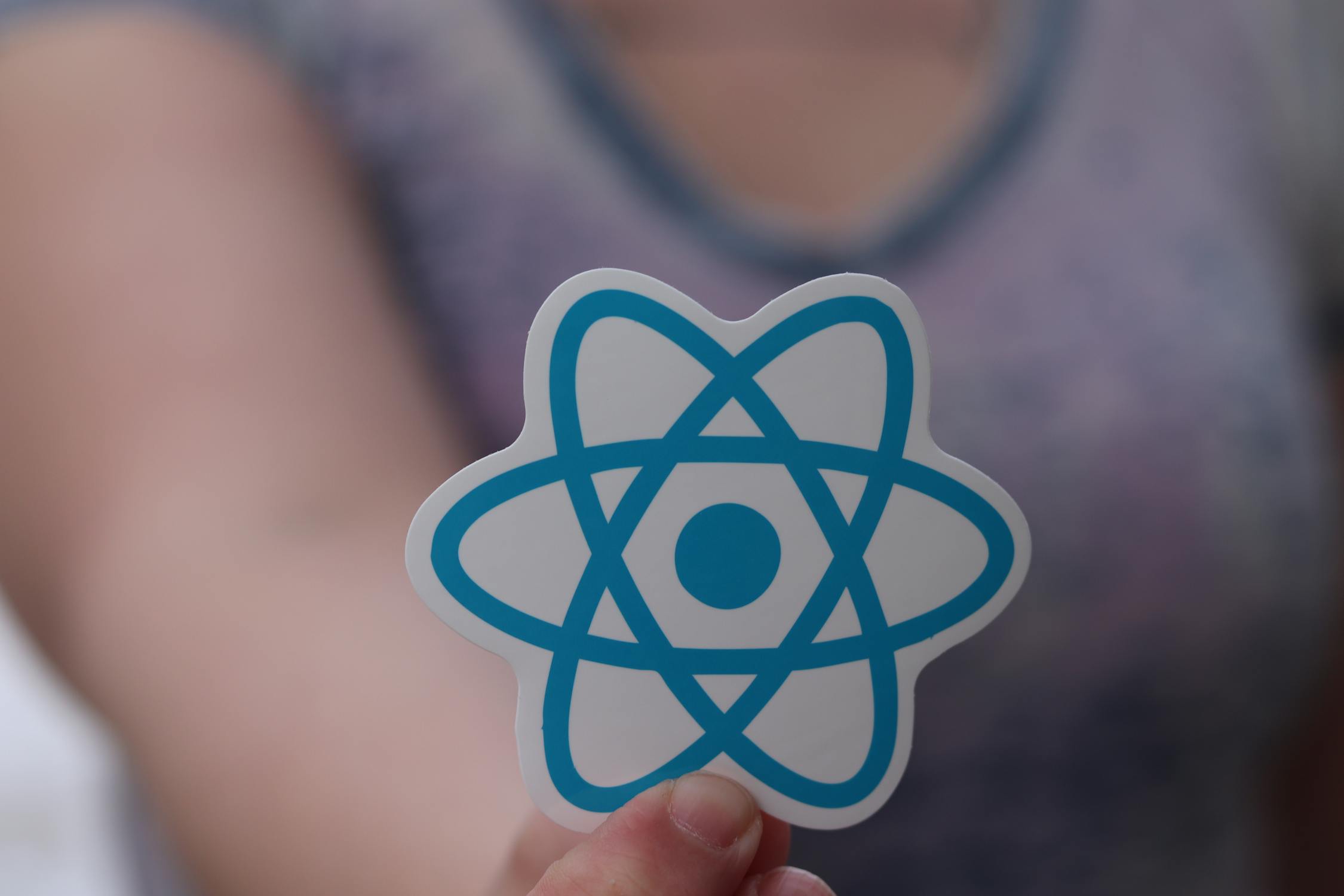
Redux Toolkit is the official, opinionated toolset for efficient Redux development. Let's explore how to use it effectively in React applications.
## Getting Started
First, install the necessary dependencies:
<pre><code class="language-bash">
npm install @reduxjs/toolkit react-redux
</code></pre>
## Creating a Store
Redux Toolkit simplifies store creation:
<pre><code class="language-typescript">
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './counterSlice';
export const store = configureStore({
reducer: {
counter: counterReducer,
},
});
</code></pre>
## Creating Slices
Slices combine reducers and actions:
<pre><code class="language-typescript">
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
},
});
</code></pre>
#react
#redux
#javascript
#web development