TypeScript
Understanding TypeScript's Type System: A Deep Dive
Explore TypeScript's powerful type system, from basic types to advanced features like conditional types and mapped types.
Obinna Aguwa •
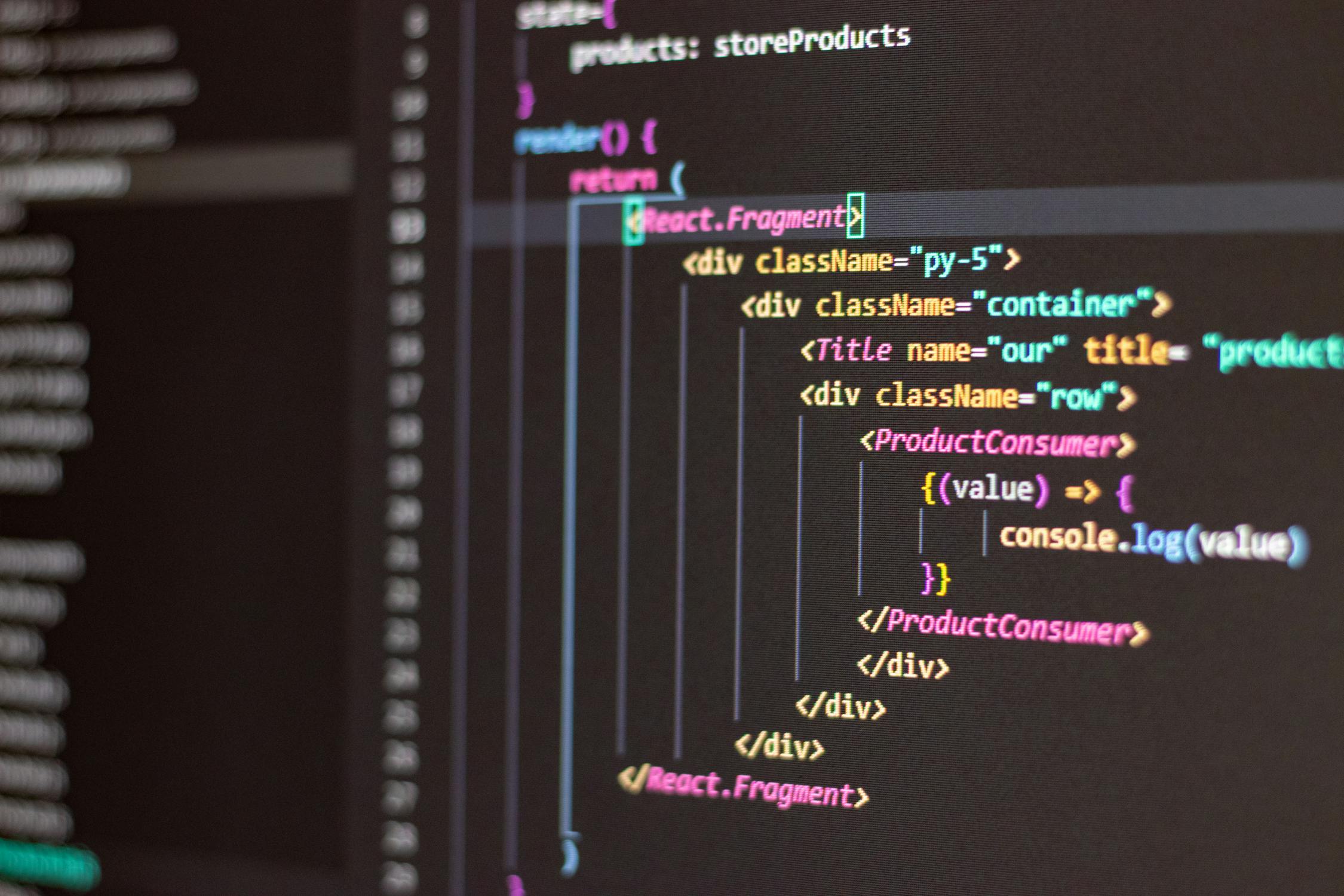
TypeScript's type system is one of its most powerful features, providing developers with tools to write safer, more maintainable code. In this article, we'll explore the fundamentals and advanced concepts of TypeScript's type system.
## Basic Types
TypeScript includes several basic types that you'll use frequently:
<pre><code class="language-typescript">
// Basic types
let isDone: boolean = false;
let decimal: number = 6;
let color: string = "blue";
let list: number[] = [1, 2, 3];
let tuple: [string, number] = ["hello", 10];
</code></pre>
## Advanced Types
TypeScript also provides advanced type features that help you create more expressive and precise types:
<pre><code class="language-typescript">
// Union Types
type StringOrNumber = string | number;
// Intersection Types
type Combined = { a: string } & { b: number };
// Type Guards
function isString(value: any): value is string {
return typeof value === "string";
}
</code></pre>
## Generic Types
Generics allow you to write flexible, reusable code:
<pre><code class="language-typescript">
function identity<T>(arg: T): T {
return arg;
}
// Usage
let output = identity<string>("myString");
</code></pre>
#typescript
#javascript
#programming
#web development